Introduction
In this tutorial I will show you how to make a basic clock in Python! Let's not waste anymore time and start coding!
Coding
Import packages
We will need to import some packages so lets import some of the following packages:
from tkinter import Tk
from tkinter import Label
import time
import sys
Tkinter will be the package for the GUI, Time will be the package to implement the time.
Creating the GUI
This is a simple 2 line for making the GUI:
master = Tk()
master.title("Digital Clock")
"Digital Clock" will be the name of application. You can change this to whatever you want.
Implementing Time
To implement time into the application we will need to tell the time package to get the time and make it into a variable for it to be placed into the GUI.
def get_time():
timeVar = time.strftime("%I:%M:%S %p")
clock.config(text=timeVar)
clock.after(200,get_time)
The 3rd line will make configurate the variable to make it text. The timeVar
is the variable for obtaining the time in your area.
Applying the time to GUI
We need to do something called label
the time into the GUI. But how do you do this? Well, we need to add some simple code like the following:
clock = Label(master, font=("Calibri",90), bg="grey",fg="white")
clock.pack()
get_time()
We just made the font Calibri at the size of 90 and the background (bg) grey and the text color white (fg). You can change these to other fonts you have installed on your computer.
Final step
The final step is to make the clock reload the time every single second. We need to do one command for the clock to do just this:
master.mainloop()
This one command to make the clock loop every second to update the time. Lets see the clock for ourself now by running it.
Of course the time will be different for you. It knows when to change to AM to PM. Customize the clock and just have fun with it!
Conclusion
That wasn't that hard making a simple digital clock in python! If you found this usful then please share this and follow me! Also check out Buy Me A Coffee!
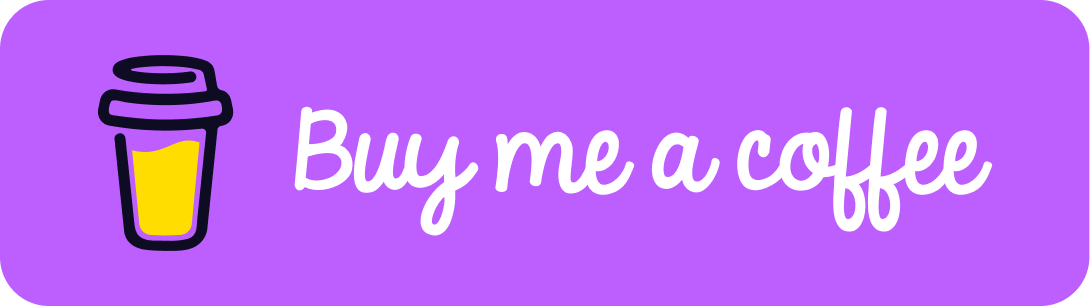